LLM Magic 🪄PHPThe missing AI Toolkit for Laravel – everything you need to build AI features, chatbots and apps
- 🎭 Multi-Provider Support – Switch between Claude, GPT-4, Gemini, and others with a single line of code. No vendor lock-in.
- 🔬 JSON Schema based – Extract structured data from unstructured content with automatic JSON schema validation and type casting.
- 🚀 Smart Document Processing – Automatically handle PDFs, images, and documents with intelligent chunking and batching. No more manual content splitting.
- 🛠️ Developer Experience Focus – Fluent interface, great IDE support, and detailed documentation. Start building in minutes, not hours.
- 🔍 Generative UI – Automatically generate UIs for your data using the same JSON schema. No manual form building.
- 🎯 Built for Laravel – Beautiful Laravel-style syntax that feels natural. Use the tools and patterns you already know and love.
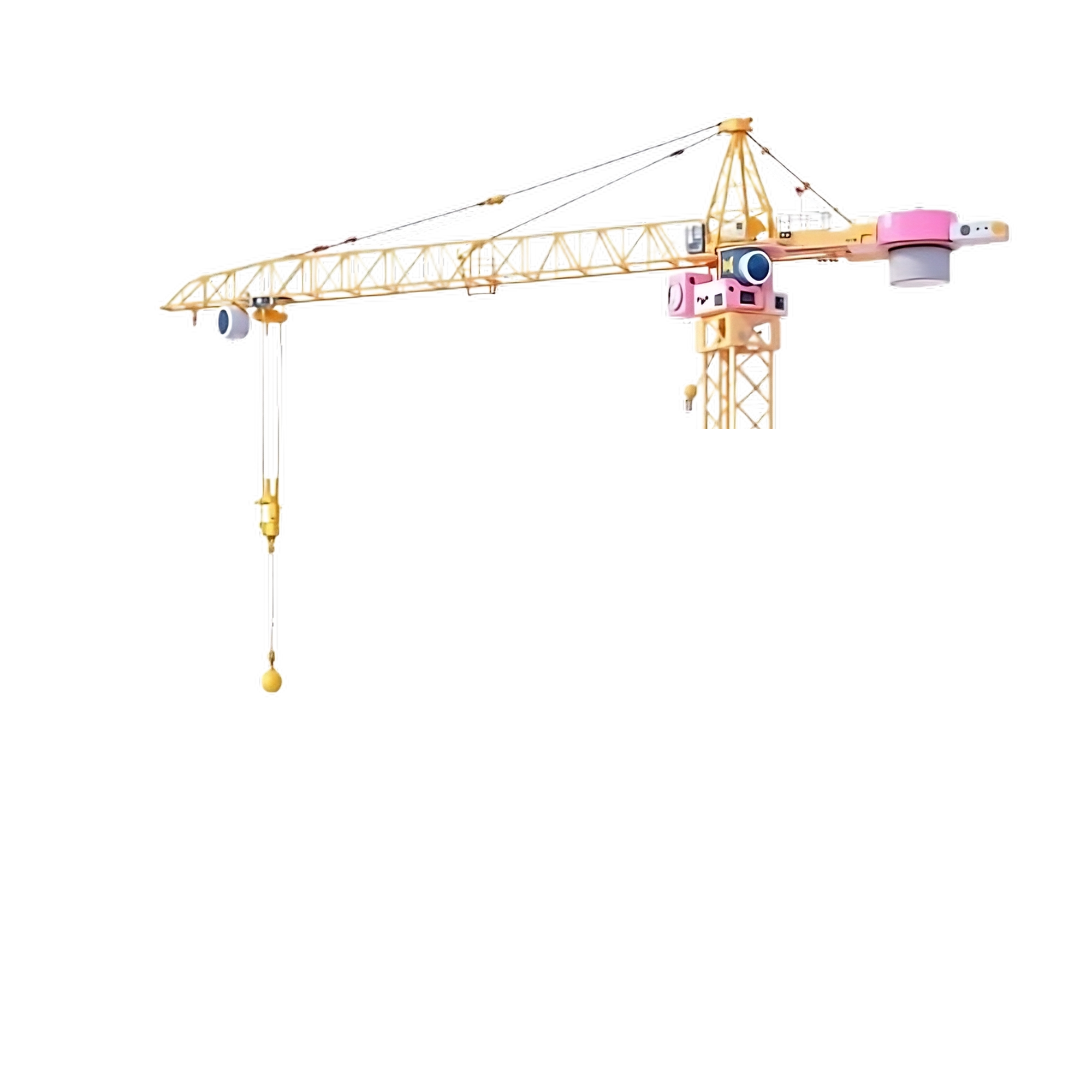
Installation
$ composer require mateffy/llm-magic
Example
$answer = Magic::chat()
->model('anthropic/claude-3.5-sonnet')
->prompt('What is the capital of France?')
->text();
// -> Paris
$invoice = Magic::extract()
->schema([
'type' => 'object',
'properties' => [
'amount' => ['type' => 'float'],
'invoice_number' => ['type' => 'string'],
],
])
->file('path/to/invoice.pdf', disk: 's3')
->send();
// -> ['amount' => 123.45, 'invoice_number' => 'INV-123']
$answer = Magic::chat()
->tools([
'add' => fn (int $a, int $b) => $a + $b,
'subtract' => fn (int $a, int $b) => $a - $b,
'multiply' => fn (int $a, int $b) => $a * $b,
'divide' => fn (int $a, int $b) => $a / $b,
])
->prompt('What is 2 + 2 - (3 * 4)?')
->lastText();
// -> I've calculated the answer to be -8.
AI Translations 🌍LaravelKeep your Laravel translations up-to-date with AI-powered translation tools
- 🌐 Automatic translation of Laravel language files
- 🔄 Translate to new languages or update existing ones without full regeneration
- 🧠 Context-aware translations
- 🔍 Smart detection of missing translations
- 💬 Interactive chat mode for translation refinement
- ✅ Validation tools for quality assurance
Installation
$ composer require --dev mateffy/ai-translations
Quickstart
$ php artisan translate
AI Translator [Source: en]
Translating 'en/validation.php'...
↳ translating 4 missing keys to 'de'...
↳ no missing translations for 'fr'...
↳ translating everything to 'es'...
...
Colorful🎨PHPType-safe color parsing, manipulating and formatting
- 🎨 Manipulate your colors with hue, lighten, alpha and similar methods
- 💅 Format your colors as Hex, RGB, HSL and more
- 👨💻 Fully typed and documented, developer focused API
- ✨ Generate new 50-950 color palettes from a single color
- 🔩 Natively supports Tailwind and Filament color definitions
Installation
$ composer require mateffy/color
Example
$color = Color::rgb(255, 0, 0)
->hue(25, add: true)
->saturation(0.5)
->alpha(0.5)
->toHex(uppercase: true, alpha: true);
Cabinet 🗄LaravelTurn-key file management, with folders and unlimited file sources
- 🔄 Laravel package unifies multiple file sources in single folder view
- 📁 Complete folder system with nesting support through dedicated database table
- 🔌 Pre-built UI components and flexible API for custom integrations
- 🎯 Unified file type system categorizes files while maintaining format specifics
- 🔗 Attach files to Laravel models with polymorphic relationships
- 🛠️ Extensible source system supports any storage type or virtual files
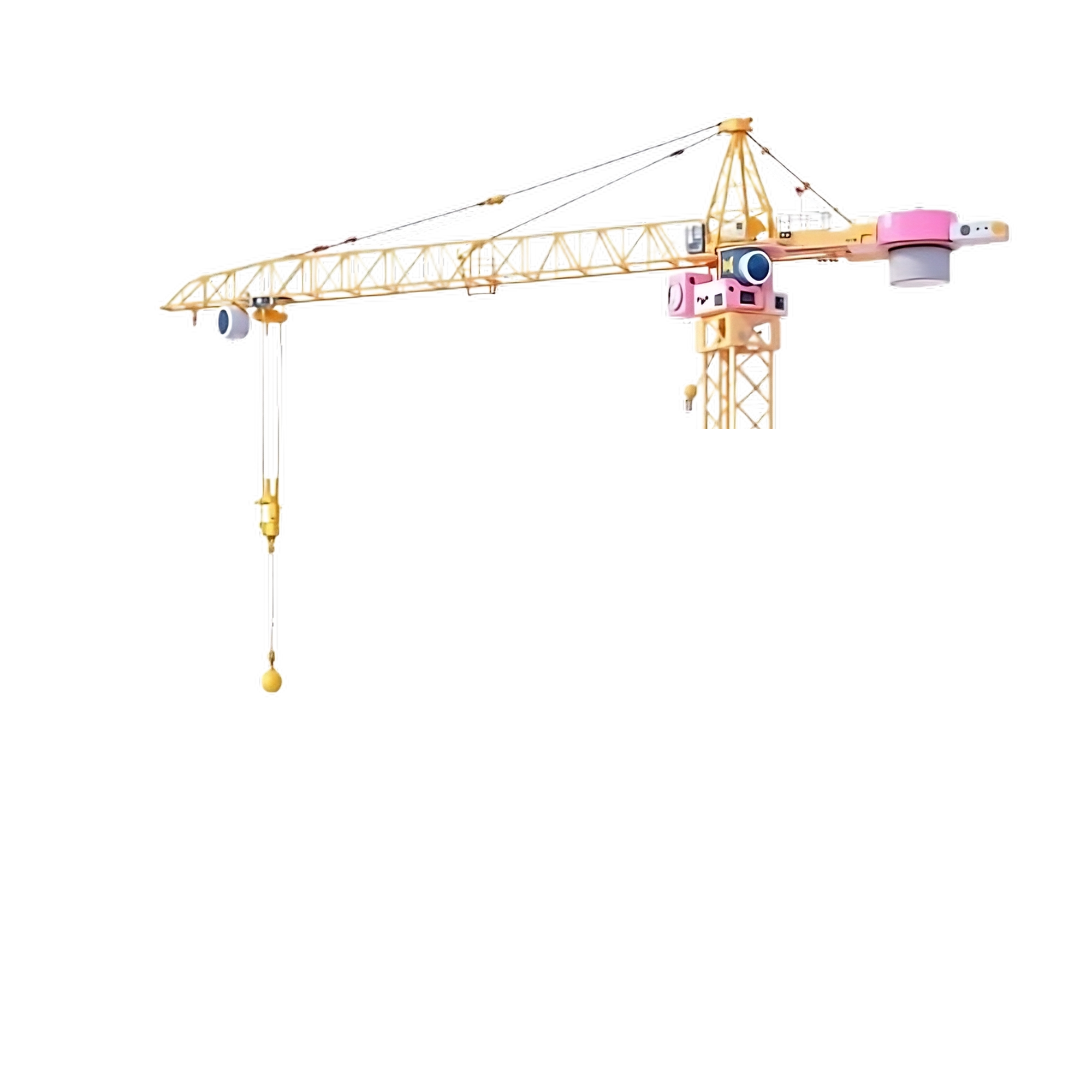
Tailpipe 🪈LaravelAccess Tailwind CSS variables in your PHP / Laravel code
- 🪈 Access Tailwind CSS variables in your PHP / Laravel code
- 📦 Generates .php file containing your theme variables
- 🔄 Uses a Tailwind plugin, so it updates on every build
- Integrations for Laravel, but can also be used in other PHP frameworks
- 🛠 Common variables are enabled by default, but you can enable more variables if you want
Installation
$ composer require mateffy/tailpipe
Example
$green500 = tailpipe('colors.green.500');
// -> #059669
$margin = tailpipe('spacing.4');
// -> 1rem
$primary = tailpipe('colors.primary.500');
// -> #your-theme-color
Why use these packages?
Powerful extensions to the TALL ecosystem
Most packages I make focus on the TALL stack: Tailwind CSS, Alpine.js, Laravel and Livewire. They are designed to work together and profit heavily from the opinionated design of the stack. Additionally, Filament is often supported as well.
Focus on developer experience
All packages solve a direct need I had during my work. They are designed to be easy to use and require as little configuration as possible. This also means they are opinionated and might not fit every use case.
Modern PHP 8+ features
All packages are written in PHP 8 and use modern features like named arguments, type hints and return types. They are also fully typed and documented with examples to make your life easier.